Capsolver: Guide to Automating CAPTCHA Solving
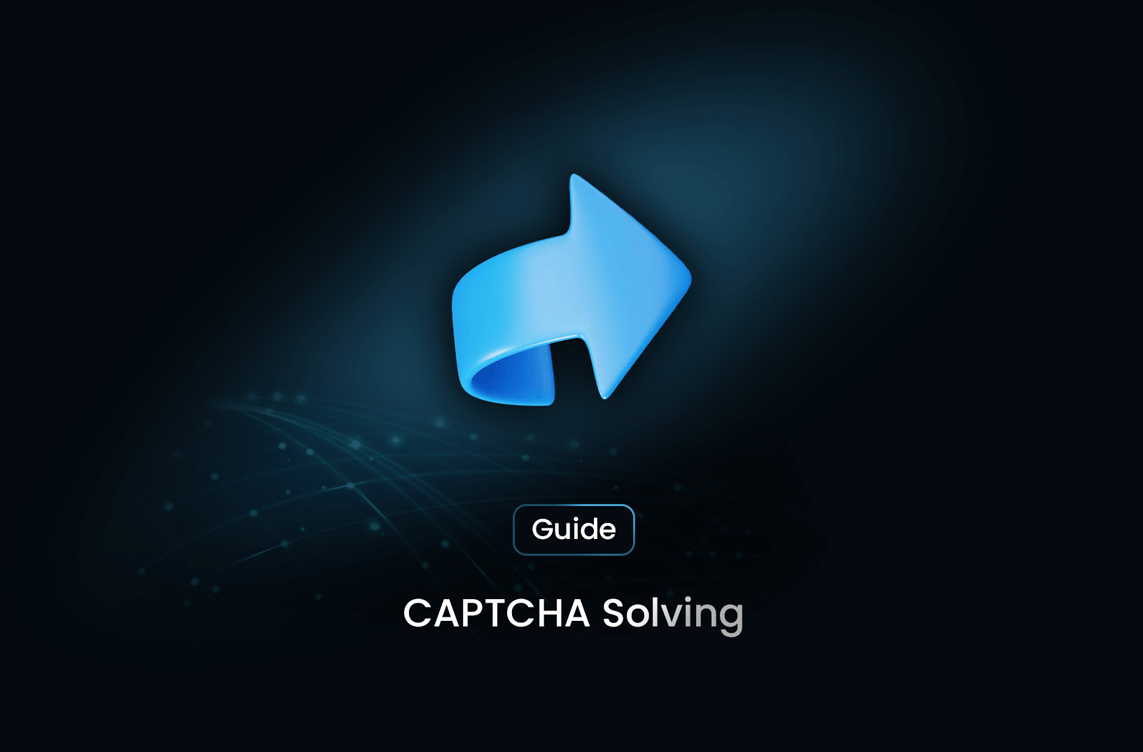
CAPTCHAs are a common challenge in web automation. While they’re essential for blocking bots, they can also be an obstacle for legitimate use cases like automated data collection or testing workflows. Capsolver simplifies this process by providing an API to handle CAPTCHA solving programmatically. In this guide, we’ll explore Capsolver, its use cases, and how to implement it with examples in Python and JavaScript.
What is Capsolver?
Capsolver is an automated CAPTCHA-solving service that helps bypass CAPTCHA challenges in various automation workflows. It supports several CAPTCHA types, including:
- reCAPTCHA v2/v3
- hCaptcha
- Image CAPTCHAs
- Audio CAPTCHAs By integrating Capsolver into your application or script, you can automate CAPTCHA-solving tasks efficiently, saving time and effort.
Why Use Capsolver?
CAPTCHA challenges disrupt automation workflows in tasks like web scraping, automated form submissions, and app testing. Capsolver eliminates this roadblock by solving CAPTCHAs programmatically.
Key Benefits:
- Scalability: Solves CAPTCHAs at scale without manual intervention.
- Versatility: Supports multiple CAPTCHA types across various platforms.
- Efficiency: Reduces latency with optimized APIs and fast response times.
Practical Use Cases
1. Web Scraping
When scraping large datasets from websites with CAPTCHA protection, Capsolver ensures uninterrupted data collection.
Example: Automating product price monitoring on e-commerce sites.
2. Automated Form Submission
Automate repetitive tasks like registering accounts or filling forms on platforms with CAPTCHA-enabled workflows.
Example: Bulk account creation for testing purposes.
3. App Testing and QA
For QA teams automating end-to-end testing, Capsolver enables seamless interaction with CAPTCHA-protected pages.
Example: Testing user registration flows without manual CAPTCHA solving.
Setting Up Capsolver
1. Create an Account
- Sign up on the Capsolver platform.
- Generate an API key from the dashboard.
2. Choose a Library
Capsolver supports multiple programming languages. For this guide, we’ll use Python and JavaScript.
How to Implement Capsolver
Python Example: Solving reCAPTCHA v2
import requests
import time
API_KEY = 'your_capsolver_api_key'
SITE_KEY = 'site_key_from_target'
URL = 'https://example.com'
# Step 1: Submit CAPTCHA for solving
response = requests.post(
'https://api.capsolver.com/solve',
json={
'clientKey': API_KEY,
'task': {
'type': 'ReCaptchaV2Task',
'websiteURL': URL,
'websiteKey': SITE_KEY
}
}
)
task_id = response.json().get('taskId')
# Step 2: Check task status
def get_solution(task_id):
while True:
result = requests.post(
'https://api.capsolver.com/getTaskResult',
json={'clientKey': API_KEY, 'taskId': task_id}
).json()
if result.get('status') == 'ready':
return result['solution']['gRecaptchaResponse']
time.sleep(5)
# Step 3: Use the CAPTCHA solution
captcha_solution = get_solution(task_id)
print(f"Solved CAPTCHA: {captcha_solution}")
JavaScript Example: Using Puppeteer and Capsolver
const puppeteer = require('puppeteer');
const fetch = require('node-fetch');
const API_KEY = 'your_capsolver_api_key';
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
// Step 1: Submit CAPTCHA task
const response = await fetch('https://api.capsolver.com/solve', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({
clientKey: API_KEY,
task: {
type: 'ReCaptchaV2Task',
websiteURL: 'https://example.com',
websiteKey: 'site_key_here',
}
})
});
const { taskId } = await response.json();
// Step 2: Wait for task result
const getSolution = async (taskId) => {
let solution;
while (!solution) {
const res = await fetch('https://api.capsolver.com/getTaskResult', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ clientKey: API_KEY, taskId })
});
const json = await res.json();
if (json.status === 'ready') solution = json.solution.gRecaptchaResponse;
else await new Promise((resolve) => setTimeout(resolve, 5000));
}
return solution;
};
const captchaSolution = await getSolution(taskId);
console.log(`Solved CAPTCHA: ${captchaSolution}`);
// Step 3: Use CAPTCHA response
await page.evaluate((response) => {
document.querySelector('#g-recaptcha-response').value = response;
}, captchaSolution);
await browser.close();
})();
Best Practices for Using Capsolver
- Efficient Task Submission: Submit tasks only when necessary to minimize API usage.
- Monitoring: Track your API usage and task success rate.
- Compliance: Always adhere to the terms of service of websites you interact with.
Troubleshooting
Common Issues
- Invalid API Key: Verify your API key in the Capsolver dashboard.
- Slow Response Times: Ensure network stability or upgrade your plan for higher priority.
- Incorrect CAPTCHA Solution: Double-check the site key and target URL in your API request.
Conclusion
Capsolver is a powerful tool for automating CAPTCHA-solving tasks, making it invaluable for web scraping, automated form submissions, and testing workflows. By integrating Capsolver with your automation scripts, you can bypass CAPTCHA challenges efficiently, allowing your applications to run uninterrupted.
If you’re looking for an even simpler and more comprehensive solution for web scraping, consider Mrscraper. With AI-powered scraping capabilities, Mrscraper handles complex tasks like data extraction and bypassing obstacles such as CAPTCHAs, making it ideal for users who want to scrape without writing code.
Whether using Capsolver directly or combining it with advanced tools like Mrscraper, you can seamlessly optimize your workflows and achieve your automation goals.
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
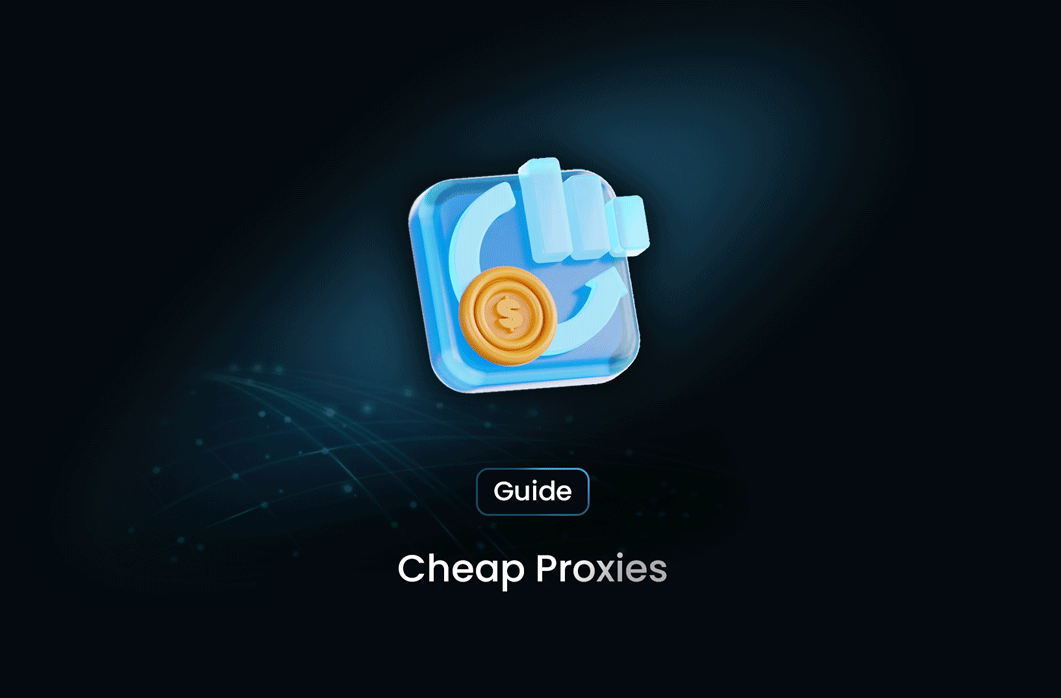
Cheap Proxies: The Best Budget-Friendly Proxy Choice
Cheap proxies are low-cost proxy servers that act as intermediaries between your device and the Internet. They provide anonymity, security, and access to restricted resources at a fraction of the cost of premium options.
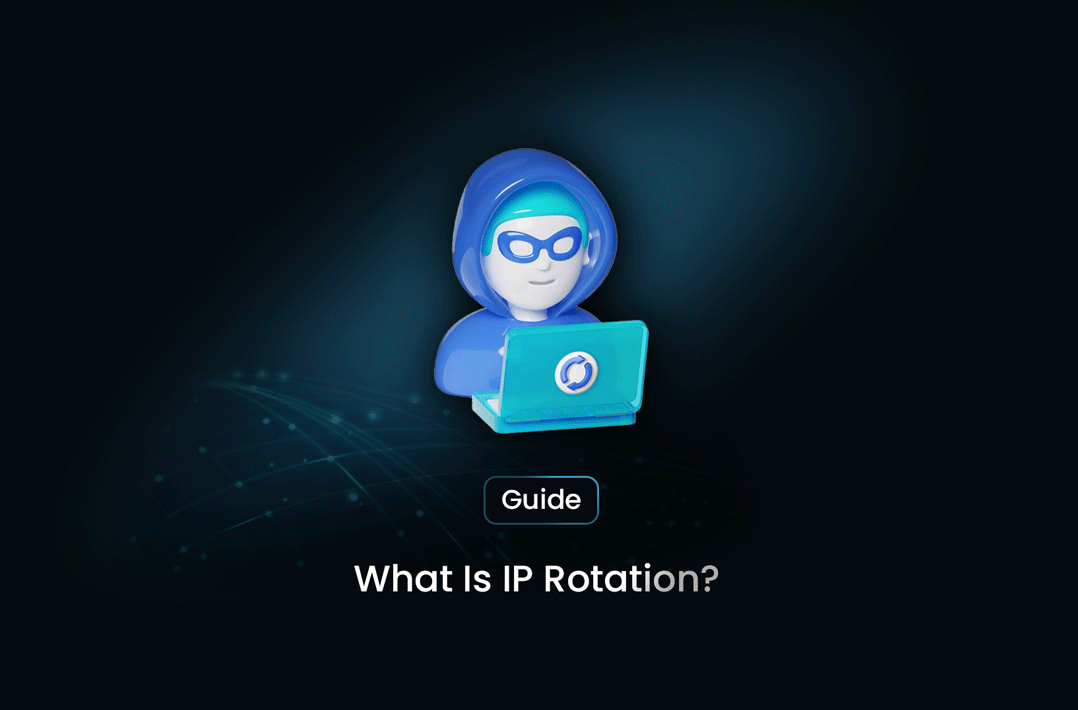
What Is IP Rotation? A Simple Guide to Staying Anonymous Online
IP rotation refers to regularly changing your IP address during online activity. This prevents websites from detecting and blocking your requests, a common issue when making frequent or automated requests to a site.
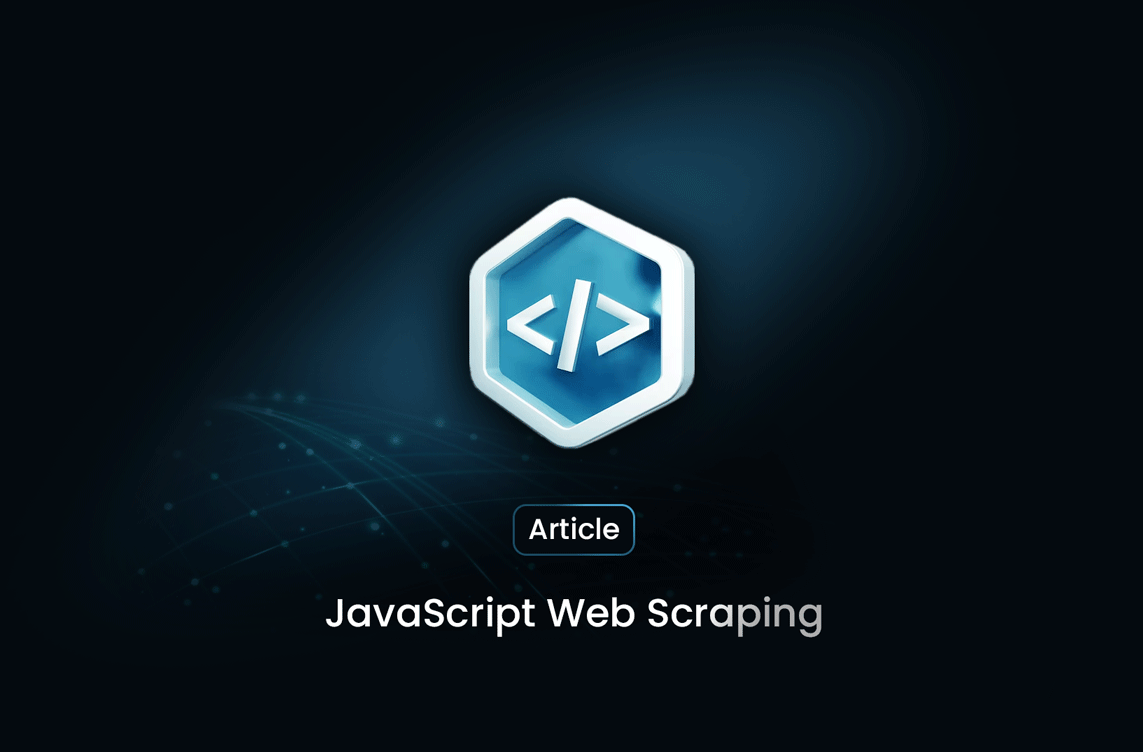
JavaScript Web Scraping
JavaScript is a great choice for web scraping with tools like Puppeteer and Cheerio for both static and dynamic sites. For more complex tasks, like bypassing CAPTCHAs or handling large-scale data, using AI-powered tools like Mrscraper can make the process easier, so you can focus on the data instead of the technical details.
@MrScraper_
@MrScraper